Introduction Why Python Interviews Matter in 2025?
Python has become the most in-demand programming language for software development, data science, and AI in 2025. Whether you’re a fresher or an experienced developer, mastering Python interview questions can significantly boost your job prospects.
Python’s Demand in 2025 – Market Insights
- Job Growth: Python-related job postings have increased by 25% YoY (Source: LinkedIn Jobs).
- High Salary: Python developers in India earn an average of ₹8-15 LPA, with experienced professionals earning over ₹25 LPA (Source: Glassdoor).
- Industry Adoption: Leading companies like Google, Netflix, Tesla, and Amazon rely on Python for AI, automation, and web development.
Why Do Interviewers Focus on Python?
Recruiters test problem-solving skills, coding efficiency, and real-world applications. Python interviews typically include:
✔ Basic & Advanced Python Concepts (Syntax, OOPs, Memory Management)
✔ Data Structures & Algorithms (Lists, Tuples, Dictionaries, Sorting)
✔ Frameworks & Libraries (Django, Flask, Pandas, NumPy)
✔ Real-World Problem-Solving (Coding Challenges & Scenario-Based Questions)
How This Blog is Curated for You?
This expert-curated list of 50 Python Interview Questions & Answers is compiled from:
✅ Industry Experts – Questions from top MNCs & startups
✅ Recent Job Interviews – Insights from Python developers
✅ Hiring Trends in 2025 – Aligned with market demand
Want to master Python interviews? 👉 Join our Python Course in Coimbatore
External Reference: Python’s Official Career Guide
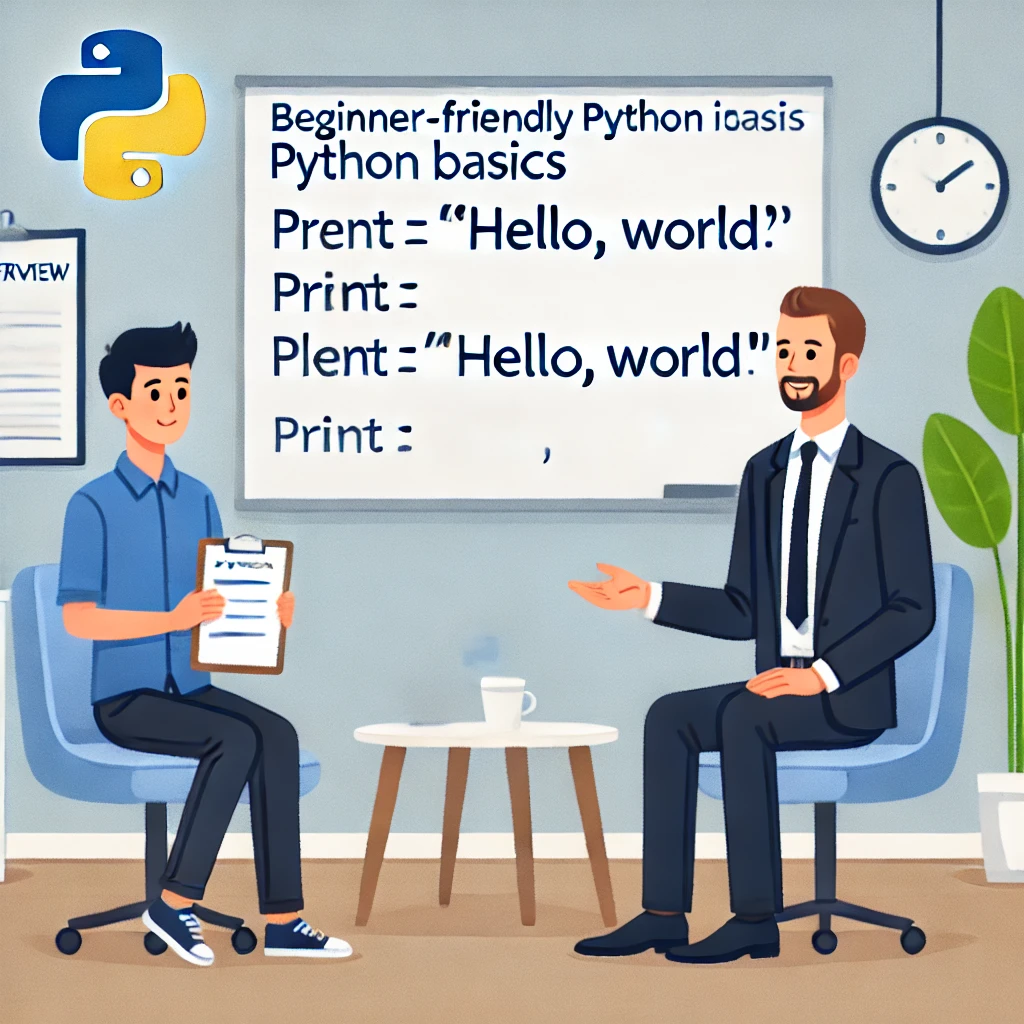
Python Interview Questions for Freshers
Python is widely used in web development, data science, AI, and automation. If you’re preparing for a Python job interview in 2025, understanding fundamental concepts is crucial. Below are the top beginner-level questions with answers to help you crack your Python interview.
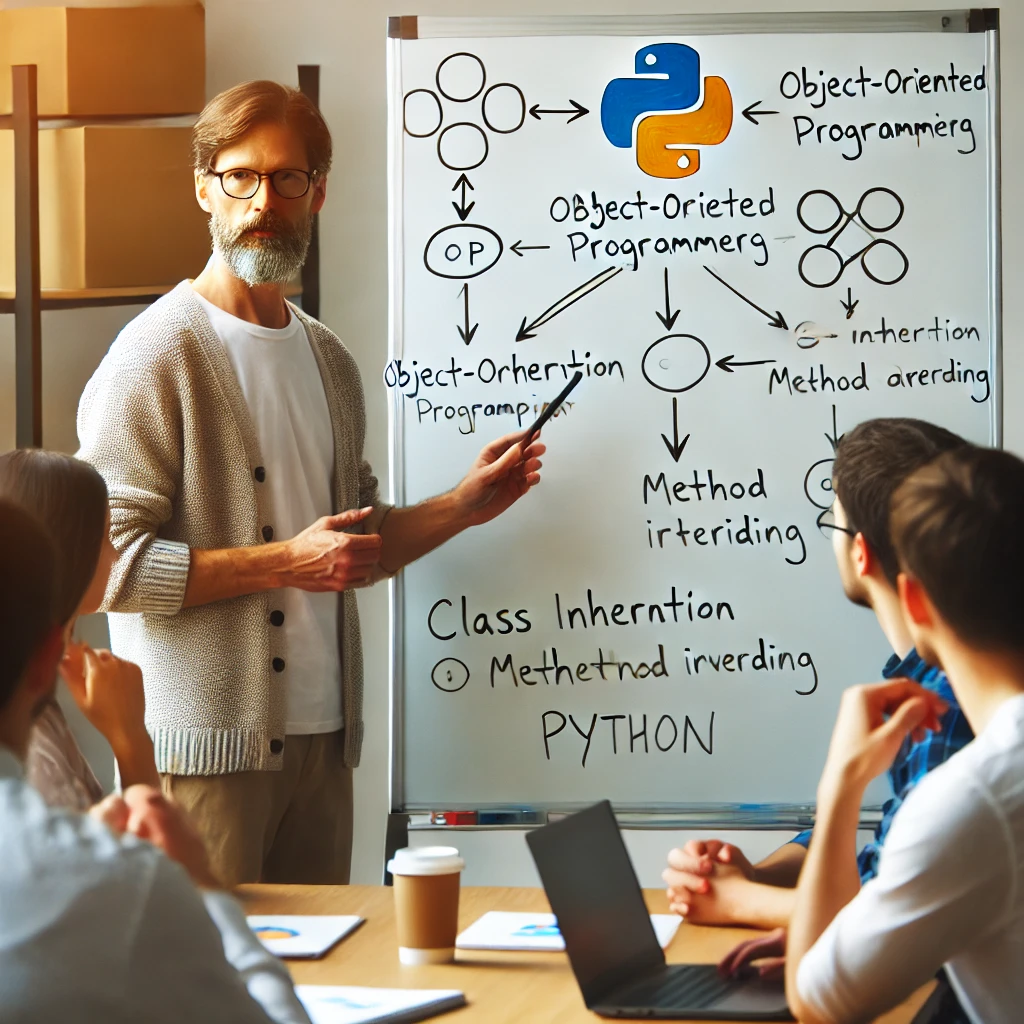
Basic Python Concepts
1. What is Python? (Real-world usage explained)
Python is an interpreted, high-level, dynamically typed programming language known for its simplicity, readability, and vast library support.
Real-world applications:
✔ Web Development – Django, Flask
✔ Data Science & AI – Pandas, NumPy, TensorFlow
✔ Automation & Scripting – DevOps, Web Scraping (Selenium, BeautifulSoup)
Further Learning: Python Course in Coimbatore
2. Python 2 vs Python 3 – Key Differences
Python 3 is the modern, supported version with better performance and syntax improvements.
Feature | Python 2 | Python 3 |
Print Statement | print "Hello" | print("Hello") |
Unicode Support | ASCII by default | Unicode by default |
Integer Division | 5/2 = 2 | 5/2 = 2.5 |
Future Support | Discontinued (since 2020) | Actively Maintained |
Further Learning: Official Python Documentation
3. What are Python’s key features? (Simple vs Advanced use cases)
✔ Easy to Learn – Simple syntax similar to English
✔ Interpreted & Dynamically Typed – No need for type declaration
✔ Large Community Support – Extensive libraries & frameworks
✔ Cross-Platform – Runs on Windows, macOS, Linux
✔ Multi-Paradigm – Supports OOP, functional & procedural programming
4. How is Python interpreted vs compiled?
- Python is an interpreted language, meaning the code is executed line-by-line rather than being compiled into machine code first.
- This makes debugging easier, but Python may run slower than compiled languages like C/C++.
Example:
python
Copy code
(
"Hello, World!")
The Python interpreter processes this line-by-line.
Further Learning: Understanding Python Internals
Data Types & Variables
5. What are Python’s built-in data types?
Python has several built-in data types, classified as:
Data Type | Examples |
Numeric | int , float , complex |
Sequence | list , tuple , range |
Text | str |
Set | set , frozenset |
Mapping | dict |
Boolean | bool (True /False ) |
Example:
python
Copy code
x =
10# int
y =
3.14# float
z =
"Python"# str
a = [
1,
2,
3]
# list
b = {
“name”:
“John”}
# dict
6. What is the difference between mutable and immutable objects?
- Mutable objects (can be changed after creation):
list
,dict
,set
- Immutable objects (cannot be changed after creation):
int
,float
,str
,tuple
Example:
python
Copy code
# Mutable list
lst = [
1,
2,
3]
lst.append(
4)
# ✅ List is modified
# Immutable tuple
tup = (
1,
2,
3)
tup[
0] =
10# ❌ TypeError: 'tuple' object does not support item assignment
7. List vs Tuple – Performance & Use Cases
Feature | List (list ) | Tuple (tuple ) |
Mutability | Mutable | Immutable |
Performance | Slower | Faster |
Memory Usage | More | Less |
Use Case | Dynamic data storage | Fixed data storage |
Example:
python
Copy code
lst = [
1,
2,
3]
# List
tup = (
1,
2,
3)
# Tuple
8. How do you swap two variables in Python? (With best practices)
Python allows variable swapping using a single line:
Example:
python
Copy code
a, b =
5,
10
a, b = b, a
print(a, b)
# Output: 10 5
Further Learning: Python Tricks for Beginners
9. Explain shallow copy vs deep copy
- Shallow Copy: Creates a new reference to the original object.
- Deep Copy: Creates a completely independent copy of the object.
Example:
python
Copy code
import copy
lst1 = [[
1,
2], [
3,
4]]
shallow_copy = copy.copy(lst1)
deep_copy = copy.deepcopy(lst1)
Further Learning: Copying in Python
10. How does Python handle memory management? (Garbage collection insights)
Python has automatic memory management using:
✔ Reference Counting – Objects are deleted when reference count reaches zero
✔ Garbage Collector – Frees unused memory (via gc
module)
Example:
python
Copy code
import gc
print(gc.get_threshold())
# Get garbage collection settings
Python Interview Questions for Experienced Professionals
For experienced professionals, Python interviews focus on OOP concepts, advanced Python features, memory management, and performance optimization. Below are key questions with answers and real-world examples.
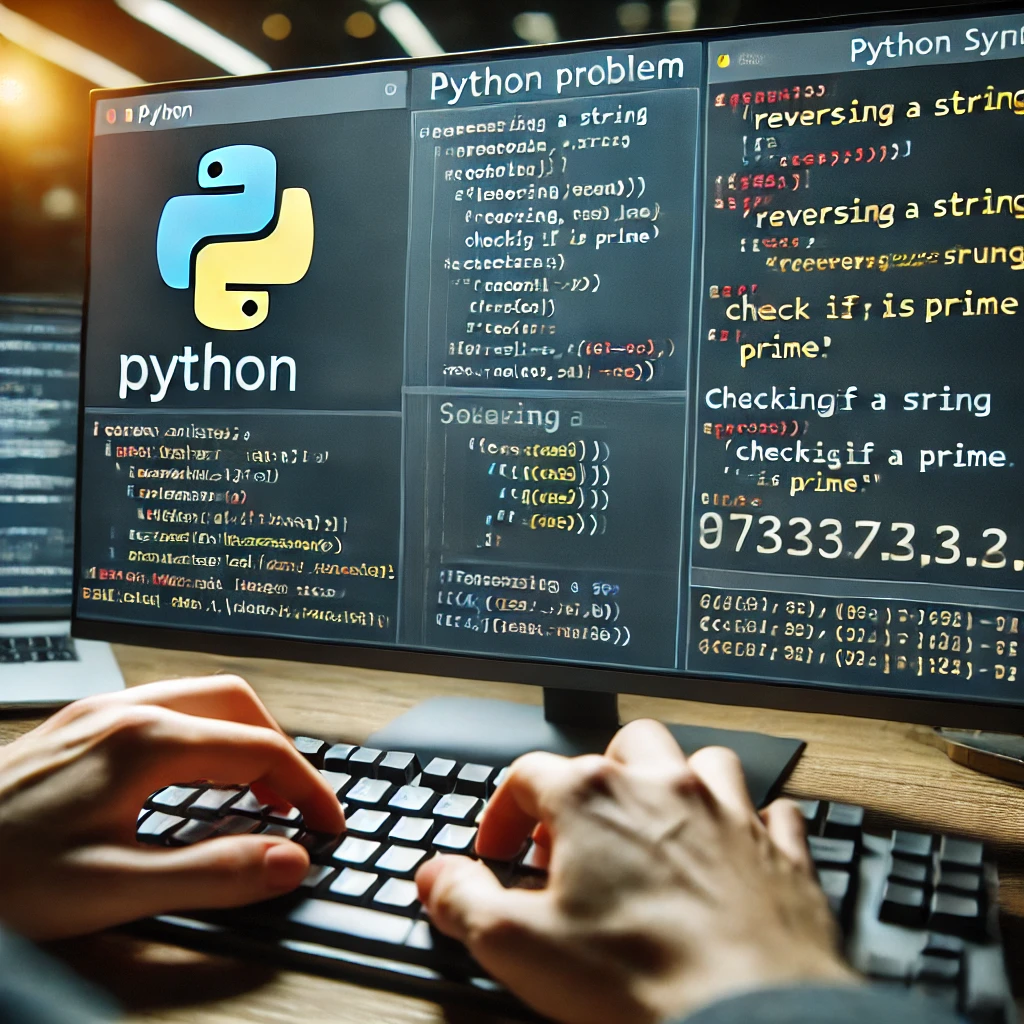
Object-Oriented Programming in Python
1. What is the difference between class and instance variables?
- Class variables are shared across all instances of a class.
- Instance variables are specific to each object.
Example:
python
Copy code
classEmployee:
company =
"TechCorp"# Class variable
def__init__
(
self, name):
self.name = name
emp1 = Employee(
"Alice")
emp2 = Employee(
"Bob")
print(emp1.company, emp1.name)
# Output: TechCorp Alice
print(emp2.company, emp2.name)
# Output: TechCorp Bob
Further Learning: Python OOP Concepts
2. Explain Python’s inheritance types with examples
Python supports 5 types of inheritance:
Inheritance Type | Example |
Single | One class inherits from another |
Multiple | A class inherits from multiple classes |
Multilevel | Derived class inherits from another derived class |
Hierarchical | Multiple classes inherit from a single base class |
Hybrid | Combination of multiple inheritance types |
Example (Multiple Inheritance):
python
Copy code
classParent1:
defmethod1
(
self):
(
"Parent1 method")
classParent2:
defmethod2
(
self):
(
"Parent2 method")
classChild(Parent1, Parent2):
obj = Child()
obj.method1()
obj.method2()
Further Learning: Python Inheritance
3. What is method overriding & method overloading in Python?
- Method Overriding: Redefining a method in a subclass.
- Method Overloading: Not directly supported, but can be implemented using *args or default parameters.
Example (Method Overriding):
python
Copy code
classParent:
defshow
(
self):
(
"Parent method")
classChild(
Parent):
defshow
(
self):
(
"Child method")
# Overriding
obj = Child()
obj.show()
Further Learning: Method Overriding in Python
4. What are metaclasses in Python?
A metaclass is a class that defines the behavior of other classes. It controls how classes are created.
Example:
python
Copy code
classMeta(
type):
def__new__
(
cls, name, bases, dct):
(
f"Creating class: {name}")
returnsuper
().__new__(cls, name, bases, dct)
classMyClass(metaclass=Meta):
pass
# Output: Creating class: MyClass
Further Learning: Python Metaclasses
5. How does Python implement encapsulation? (With Code Example)
Encapsulation is achieved using private (__var
), protected (_var
), and public (var
) attributes.
Example:
python
Copy code
classBankAccount:
def__init__
(
self, balance):
self.__balance = balance
defdeposit
(
self, amount):
self.__balance += amount
defget_balance
(
self):
return
self.__balance
acc = BankAccount(
1000)
print(acc.get_balance())
# Output: 1000
print(acc.__balance)
# AttributeError (Private variable)
Advanced Python Concepts
6. What is a Python generator?
A generator is a special type of iterator that allows lazy evaluation using yield
.
Example:
python
Copy code
defcount():
n =
while
n <=
5:
yield
n
n +=
gen = count()
(
next(gen))
# Output: 1
(
next(gen))
# Output: 2
7. Explain Python’s GIL (Global Interpreter Lock) and its impact on multithreading
- GIL allows only one thread to execute Python bytecode at a time, limiting multi-threading performance.
- Use multiprocessing for CPU-bound tasks instead of threading.
Example (Multiprocessing instead of Threading):
python
Copy code
frommultiprocessing
importPool
defwork(
n):
return
n * n
withPool(
5)
asp:
(p.
map(work, [
1,
2,
3,
4,
5]))
# Output: [1, 4, 9, 16, 25]
8. How does Python handle exceptions? (Best practices for error handling)
Python uses try-except-finally
for error handling.
📌 Example:
python
Copy code
try:
result =
10/
0
exceptZeroDivisionError
ase:
(
f"Error: {e}")
# Output: Error: division by zero
finally:
(
"Execution completed.")
Further Learning: Python Exception Handling
9. What are Python decorators, and how do they work?
Decorators are functions that modify another function’s behavior.
📌 Example:
python
Copy code
defdecorator(
func):
defwrapper
():
(
"Before function call")
func()
(
"After function call")
return
wrapper
@decorator
defhello():
(
"Hello, Python!")
hello()
10. What is monkey patching in Python? (Use Cases & Risks)
- Monkey Patching dynamically modifies a class or module at runtime.
- Risk: It can break dependencies and lead to unexpected behavior.
Example:
python
Copy code
classA:
defshow
(
self):
(
"Original Method")
defnew_show():
(
"Patched Method")
A.show = new_show
obj = A()
obj.show()
# Output: Patched Method
Python Coding Interview Questions (With Solutions)
Python coding interviews often test problem-solving, logic, and algorithm implementation skills. Here are 5 commonly asked Python coding interview questions with solutions and best practices.
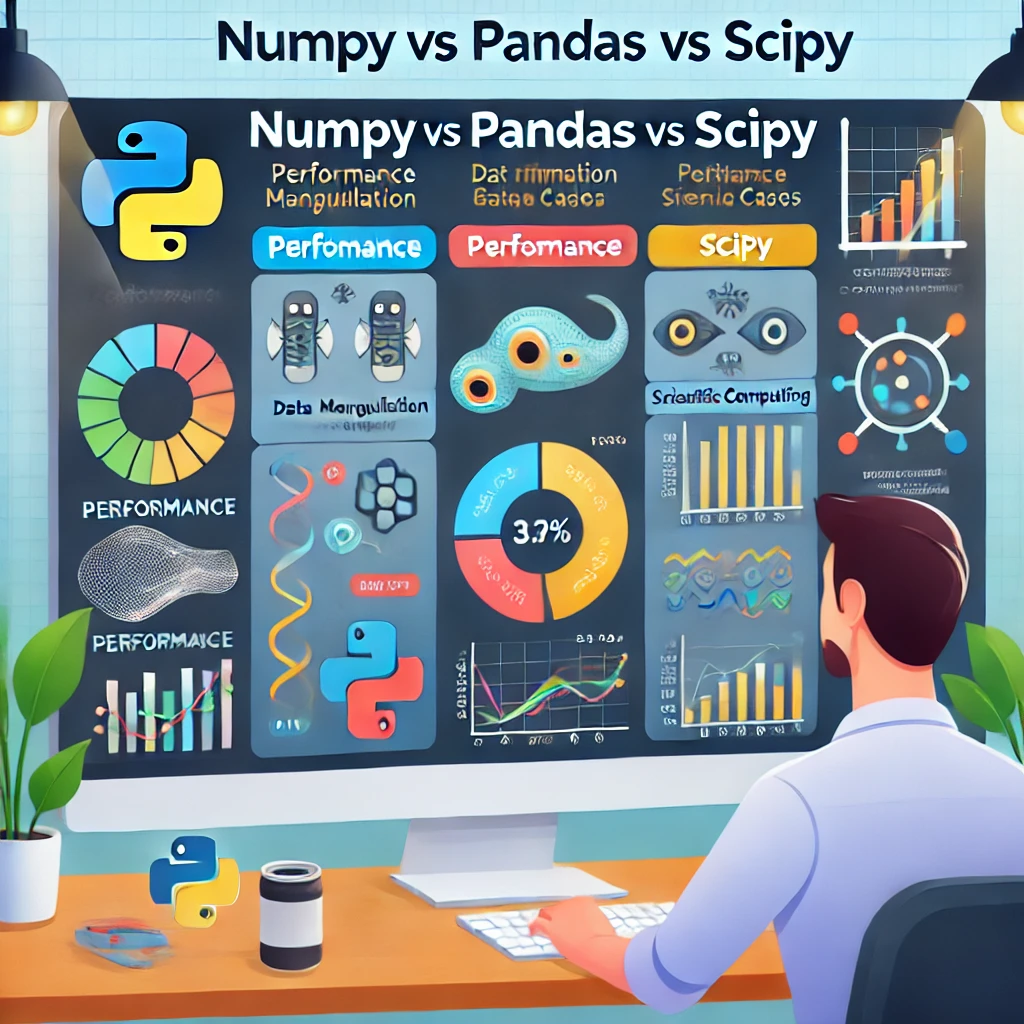
1. Write a Python program to reverse a string
Using slicing ([::-1]
) is the simplest approach, but we can also use loops or recursion.
Example (Using Slicing):
python
Copy code
defreverse_string
(
s):
return
s[::-
1]
(reverse_string(
"Python"))
# Output: nohtyP
Example (Using Loop):
python
Copy code
defreverse_string
(
s):
reversed_str =
""
for
char
ins:
reversed_str = char + reversed_str
return
reversed_str
(reverse_string(
"Python"))
# Output: nohtyP
Example (Using Recursion):
python
Copy code
defreverse_string
(
s):
if
len
(s) ==
0:
return
s
return
s[-
1] + reverse_string(s[:-
1])
(reverse_string(
"Python"))
# Output: nohtyP
Further Learning: Python String Methods
2. Implement a function to check if a number is prime
A prime number is only divisible by 1 and itself.
Example (Efficient Method):
python
Copy code
defis_prime
(
n):
if
n <
2:
return
False
for
i
inrange
(
2,
int(n **
0.5) +
1):
if
n % i ==
0:
return
False
return
True
(is_prime(
29))
# Output: True
(is_prime(
10))
# Output: False
Optimization:
- Only check divisibility up to
sqrt(n)
, reducing time complexity to O(√n). - Avoid even numbers except
2
for optimization.
3. Find the second-largest element in a list using Python
We can solve this using sorting, loops, or the heapq
module.
Example (Using Sorting – Simple but Inefficient)
python
Copy code
defsecond_largest
(
lst):
unique_nums =
list(
set(lst))
# Remove duplicates
unique_nums.sort()
return
unique_nums[-
2]
iflen
(unique_nums) >
1else
None
(second_largest([
10,
20,
4,
45,
99,
99]))
# Output: 45
Example (Using Loop – More Efficient, O(n) Time Complexity)
python
Copy code
defsecond_largest
(
lst):
first = second =
float(
'-inf')
for
num
inlst:
if
num > first:
second, first = first, num
elif
num > second
andnum != first:
second = num
return
second
ifsecond !=
float(
'-inf')
elseNone
(second_largest([
10,
20,
4,
45,
99,
99]))
# Output: 45
Further Learning: Python Lists & Sorting
4. Write a Python program to check if a string is a palindrome
A palindrome reads the same forward and backward.
Example (Using Slicing – Most Pythonic Way)
python
Copy code
defis_palindrome
(
s):
return
s == s[::-
1]
(is_palindrome(
"radar"))
# Output: True
(is_palindrome(
"python"))
# Output: False
Example (Using Two-Pointer Technique – More Efficient)
python
Copy code
defis_palindrome
(
s):
left, right =
0,
len(s) -
1
while
left < right:
if
s[left] != s[right]:
return
False
left +=
1
right -=
1
return
True
(is_palindrome(
"radar"))
# Output: True
(is_palindrome(
"python"))
# Output: False
Further Learning: Python String Handling
5. Implement a Fibonacci series using recursion
The Fibonacci series follows the pattern: 0, 1, 1, 2, 3, 5, 8, 13...
where:
F(0) = 0, F(1) = 1
F(n) = F(n-1) + F(n-2) for n ≥ 2
Example (Using Recursion – Inefficient O(2^n) Time Complexity)
python
Copy code
deffibonacci
(
n):
if
n <=
0:
return
0
elif
n ==
1:
return
1
return
fibonacci(n -
1) + fibonacci(n -
2)
([fibonacci(i)
fori
inrange
(
10)])
# Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Example (Using Memoization – Optimized O(n) Time Complexity)
python
Copy code
deffibonacci_memo
(
n, memo={}):
if
n
inmemo:
return
memo[n]
if
n <=
0:
return
0
elif
n ==
1:
return
1
memo[n] = fibonacci_memo(n -
1, memo) + fibonacci_memo(n -
2, memo)
return
memo[n]
([fibonacci_memo(i)
fori
inrange
(
10)])
# Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Example (Using Iteration – Best Performance O(n))
python
Copy code
deffibonacci_iterative
(
n):
a, b =
0,
1
for
_
inrange
(n):
a, b = b, a + b
return
a
([fibonacci_iterative(i)
fori
inrange
(
10)])
# Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Python Libraries & Frameworks Interview Questions
Python has a rich ecosystem of libraries and frameworks for data science, web development, and system-level programming. Below are common interview questions with answers, covering NumPy, Pandas, Django, Flask, FastAPI, and logging.
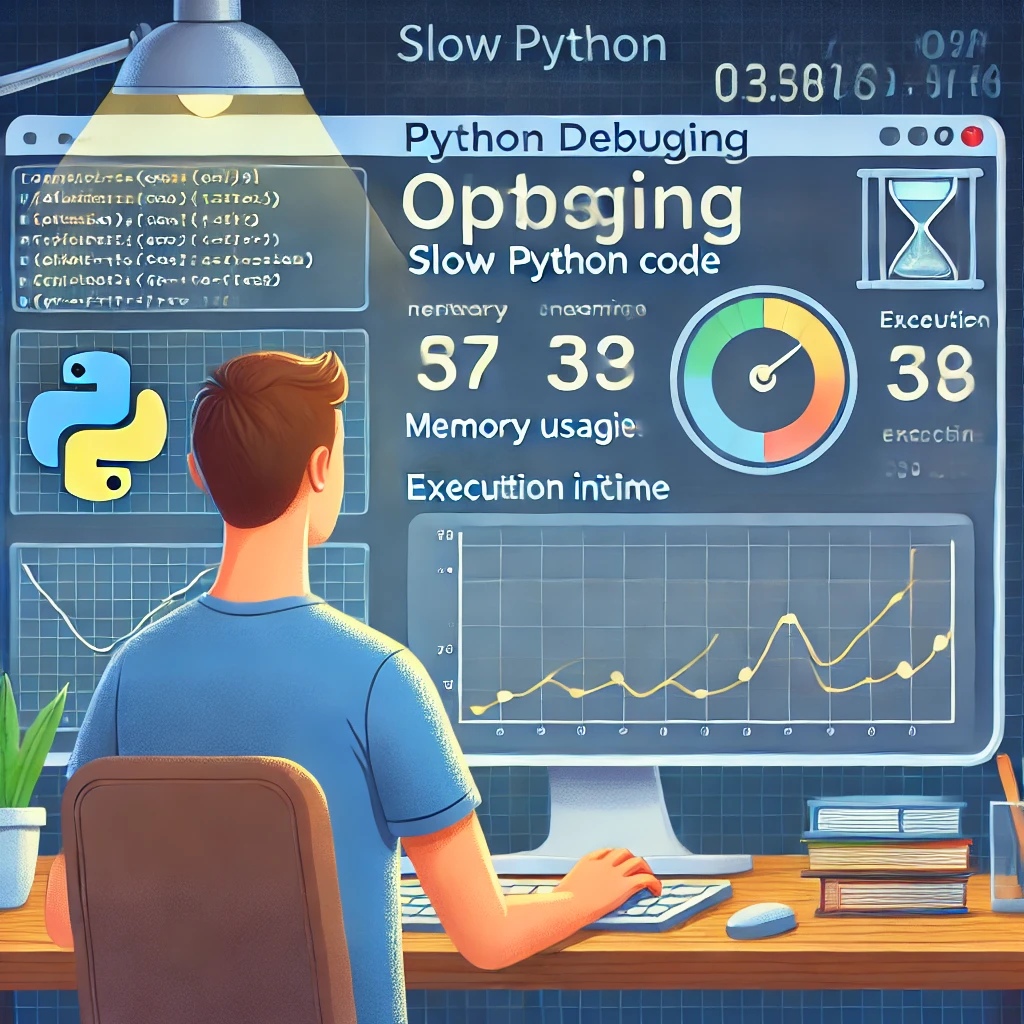
1. What is NumPy, and why is it important?
NumPy (Numerical Python) is a powerful library for numerical computing in Python. It provides multi-dimensional arrays, mathematical functions, and linear algebra operations, making it a core tool in data science and machine learning.
Real-World Use Cases:
- Data Science & ML: Used for data preprocessing and mathematical operations.
- Scientific Computing: Efficient handling of large datasets.
- Image Processing: Used in OpenCV for matrix operations on images.
2. Explain the difference between Pandas DataFrame and NumPy array
Both Pandas and NumPy are used for handling structured data, but they serve different purposes.
Feature | NumPy Array | Pandas DataFrame |
Data Type | Homogeneous (same type) | Heterogeneous (mixed types) |
Indexing | Integer-based | Label & Integer-based |
Performance | Faster | Slightly slower due to additional functionality |
Functionality | Basic numerical operations | Advanced data manipulation, filtering, and analysis |
Use Cases | Machine Learning, Mathematical Computing | Data Analysis, Business Intelligence |
Example:
python
Copy code
importnumpy
asnp
importpandas
aspd
arr = np.array([
1,
2,
3,
4])
# NumPy Array
df = pd.DataFrame({
'A': [
1,
2,
3],
'B': [
'X',
'Y',
'Z']})
# Pandas DataFrame
print(arr)
print(df)
3. What are the key differences between Django and Flask?
Both Django and Flask are Python web frameworks, but they differ in their philosophy, scalability, and use cases.
Feature | Django | Flask |
Type | Full-stack framework | Micro-framework |
Built-in Features | Authentication, ORM, Admin Panel | Minimal, extendable with libraries |
Learning Curve | Steeper | Easier |
Best for | Large-scale applications | Small to medium-sized apps |
Flexibility | Less, but robust structure | More, but requires manual setup |
Use Cases:
- Django: E-commerce sites, large-scale CMS platforms.
- Flask: Small web applications, REST APIs.
4. How does FastAPI compare to Flask for building APIs?
FastAPI is a modern asynchronous web framework designed for building APIs faster and more efficiently than Flask.
Feature | FastAPI | Flask |
Performance | Faster (async support) | Slower (synchronous) |
Type Checking | Built-in Pydantic validation | Manual validation |
Asynchronous Support | Yes | Requires additional setup |
Learning Curve | Moderate | Easy |
Best for | High-performance APIs, microservices | Simple APIs |
Example (FastAPI vs Flask API Setup):
FastAPI:
python
Copy code
fromfastapi
importFastAPI
app = FastAPI()
@app.get("/")
defread_root():
return
{
"message":
"Hello, FastAPI"}
Flask:
python
Copy code
fromflask
importFlask
app = Flask(__name__)
@app.route("/")
defhome():
return
{
"message":
"Hello, Flask"}
5. Explain logging in Python – Why is it important?
Logging is essential for debugging, monitoring, and maintaining Python applications. Instead of using print()
, logging provides structured and configurable logs.
Best Practices:
- Use different logging levels (
DEBUG
,INFO
,WARNING
,ERROR
,CRITICAL
). - Write logs to files instead of printing to the console.
- Use log rotation to manage log file size.
Example (Basic Logging Setup):
python
Copy code
import logging
# Configure logging
logging.basicConfig(level=logging.INFO, filename=
'app.log',
format=
'%(asctime)s - %(levelname)s - %(message)s')
# Log messages
logging.info(
"Application started")
logging.warning(
"This is a warning")
logging.error(
"An error occurred")
Further Learning: Python Logging Module
Python Scenario-Based & Real-World Interview Questions
Python developers often face real-world challenges that require optimization, debugging, security, and memory management. Below are scenario-based interview questions with expert solutions to help you crack your next Python interview!
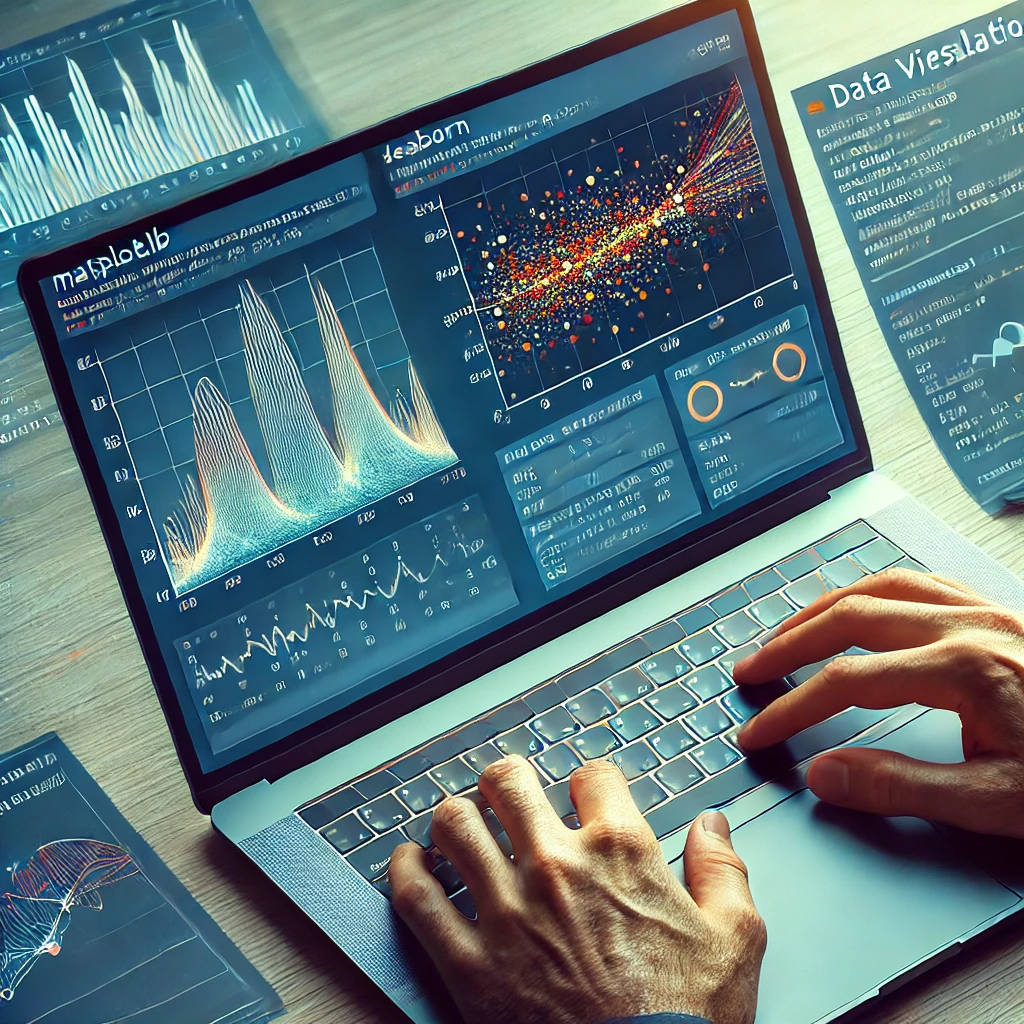
1. How would you optimize a slow-running Python program?
A slow-running Python program can be optimized using various techniques:
Optimization Strategies:
- Use Built-in Functions & Libraries: Native functions like
sum()
,map()
, andzip()
are optimized in C. - Use List Comprehensions Instead of Loops: Faster than traditional loops.
- Use Generators Instead of Lists: Saves memory when working with large data.
- Leverage Multi-threading & Multi-processing: Helps in CPU-bound and I/O-bound tasks.
- Profile Your Code: Use
cProfile
to identify bottlenecks.
Example (Using Generators for Optimization):
python
Copy code
deflarge_numbers
():
for
i
inrange
(
10**
6):
# Generates 1 million numbers without storing them in memory
yield
i
gen = large_numbers()
(
next(gen))
# Fetches numbers on demand
Further Learning: Python Performance Optimization
2. How do you prevent memory leaks in Python applications?
Python uses automatic garbage collection, but circular references and global variables can cause memory leaks.
Best Practices to Prevent Memory Leaks:
- Use Weak References (
weakref
module) to prevent reference cycles. - Use context managers (
with
statement) for file handling. - Delete unused objects using
del
and callgc.collect()
when needed. - Avoid using global variables for large data structures.
Example (Using Weak References):
python
Copy code
import weakref
classData
:
pass
obj = Data()
weak_obj = weakref.ref(obj)
# Weak reference prevents memory leaks
(weak_obj())
# Access the object
del obj
(weak_obj())
# Now returns None (no memory leak)
Further Learning: Python Garbage Collection
3. How do you handle large files efficiently in Python?
Handling large files in Python requires streaming and memory-efficient techniques.
Best Practices for Large Files:
- Read files in chunks instead of loading everything into memory.
- Use memory-mapped files (
mmap
) for efficient processing. - Use Pandas’
chunksize
for large CSV files.
Example (Reading Large Files in Chunks):
python
Copy code
defread_large_file
(
filename):
with
open
(filename,
"r")
asfile:
for
line
infile:
# Reads line by line without loading entire file
(line.strip())
read_large_file(
“big_data.txt”)
5. What are the security best practices in Python applications?
Security is crucial in Python applications, especially for web development and API security.
Security Best Practices:
- Avoid hardcoded secrets (Use
.env
files instead). - Sanitize user input to prevent SQL Injection & XSS.
- Use parameterized queries in database interactions.
- Validate API requests to prevent unauthorized access.
- Implement proper error handling instead of exposing sensitive data.
Example (Using Environment Variables for Secrets):
python
Copy code
import os
fromdotenv
importload_dotenv
load_dotenv()
# Load environment variables
API_KEY = os.getenv(
"API_SECRET_KEY")
# Securely fetch API key
(API_KEY)
# Never hardcode API keys!
5. How would you debug a complex Python script? (Step-by-step approach)
Debugging is a critical skill for Python developers. The best debugging approach involves logging, breakpoints, and profiling.
Step-by-Step Debugging Guide:
- Use
print()
wisely: Start by printing variables at key points. - Use
logging
instead ofprint()
: Structured logs help track issues. - Use Debuggers (
pdb
& VS Code Debugger): Pause execution & inspect variables. - Use
cProfile
to analyze performance: Find slow-running functions. - Write Unit Tests: Catch issues early.
Example (Using pdb
for Debugging):
python
Copy code
import pdb
defbuggy_function
():
x =
10
y =
0
pdb.set_trace()
# Start Debugger
return
x / y
# Causes ZeroDivisionError
buggy_function()
Python Data Science & Machine Learning Interview Questions
Python is the dominant language in Data Science and Machine Learning due to its extensive libraries and ease of use. Below are key Python interview questions related to Data Science, ML, and large-scale data processing, along with expert answers.
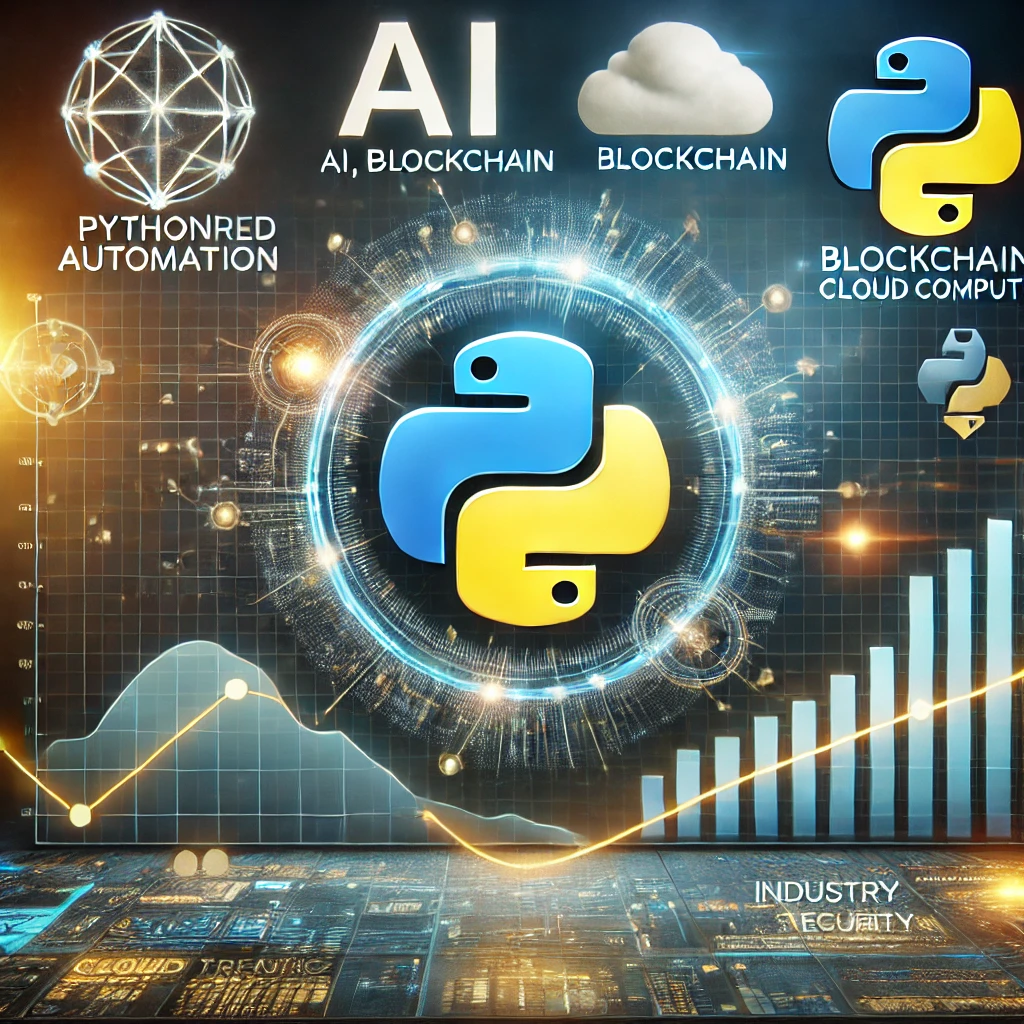
1. What is the role of Python in Data Science?
Python plays a crucial role in Data Science due to its simplicity, vast libraries, and community support.
Why Python for Data Science?
- Rich Libraries: NumPy, Pandas, Scikit-learn, TensorFlow, PyTorch.
- Data Manipulation & Analysis: Pandas for structured data, NumPy for arrays.
- Machine Learning & AI: Scikit-learn for ML, TensorFlow for Deep Learning.
- Data Visualization: Matplotlib, Seaborn for charts & graphs.
- Big Data Integration: Works with Apache Spark, Hadoop, and Dask.
Further Learning: Python for Data Science
2. Explain the difference between supervised and unsupervised learning.
Supervised and unsupervised learning are two fundamental categories of machine learning.
Key Differences:
Feature | Supervised Learning | Unsupervised Learning |
Data Type | Labeled Data (X, Y) | Unlabeled Data (Only X) |
Goal | Predict output based on input | Identify patterns & clusters |
Examples | Classification, Regression | Clustering, Anomaly Detection |
Algorithms | Linear Regression, Decision Trees, SVM | K-Means, PCA, DBSCAN |
Example:
Supervised Learning: Predicting house prices based on historical data.
Unsupervised Learning: Identifying customer segments for marketing.
3. What are the most commonly used Python libraries for ML?
Machine Learning in Python is powered by various libraries:
Popular ML Libraries:
- Scikit-learn: Classical ML algorithms (Regression, Classification).
- TensorFlow & PyTorch: Deep Learning & Neural Networks.
- XGBoost & LightGBM: Boosting algorithms for tabular data.
- NLTK & SpaCy: Natural Language Processing (NLP).
- OpenCV: Image Processing & Computer Vision.
Example (Using Scikit-learn for a Simple Model):
python
Copy code
fromsklearn.linear_model
importLogisticRegression
fromsklearn.datasets
importload_iris
fromsklearn.model_selection
importtrain_test_split
# Load dataset
X, y = load_iris(return_X_y=
True)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=
0.2)
# Train model
model = LogisticRegression()
model.fit(X_train, y_train)
# Predict
print(model.score(X_test, y_test))
4. What is the difference between NumPy and SciPy?
Both NumPy and SciPy are essential for scientific computing in Python.
Key Differences:
Feature | NumPy | SciPy |
Purpose | Handles arrays, matrices | Advanced scientific computations |
Core Functionality | Linear algebra, array operations | Statistics, Signal Processing, Optimization |
Dependencies | Foundation library | Built on NumPy |
Example Use | Creating arrays | Solving differential equations |
Example (Using NumPy for Array Operations):
python
Copy code
importnumpy
asnp
a = np.array([
1,
2,
3])
b = np.array([
4,
5,
6])
print(np.dot(a, b))
# Dot product of vectors
Example (Using SciPy for Optimization):
python
Copy code
fromscipy.optimize
importminimize
defobjective(
x):
return
x**
2+
5*x +
4
result = minimize(objective,
0)
# Find minimum
print(result.x)
Further Learning: NumPy vs SciPy
5. How does Python handle large-scale data processing? (Real-world applications)
Python efficiently handles big data and large-scale machine learning through distributed computing.
Techniques for Handling Large Data:
- Dask & Vaex: Scales Pandas operations for big data.
- Apache Spark (PySpark): Distributed data processing.
- HDF5 & Parquet Files: Store large datasets efficiently.
- Cloud Integration: AWS S3, Google BigQuery for data storage.
Example (Using Dask for Large Data Processing):
python
Copy code
importdask.dataframe
asdd
df = dd.read_csv(
"large_data.csv")
# Reads large CSV efficiently
print(df.head())
# Fetches first few rows
Further Learning: Handling Large Data in Python
Latest Python Trends & Future Scope in 2025
Python continues to be a dominant language in AI, Blockchain, Cloud Computing, and Web Development. Below are the latest trends and future insights backed by industry reports.
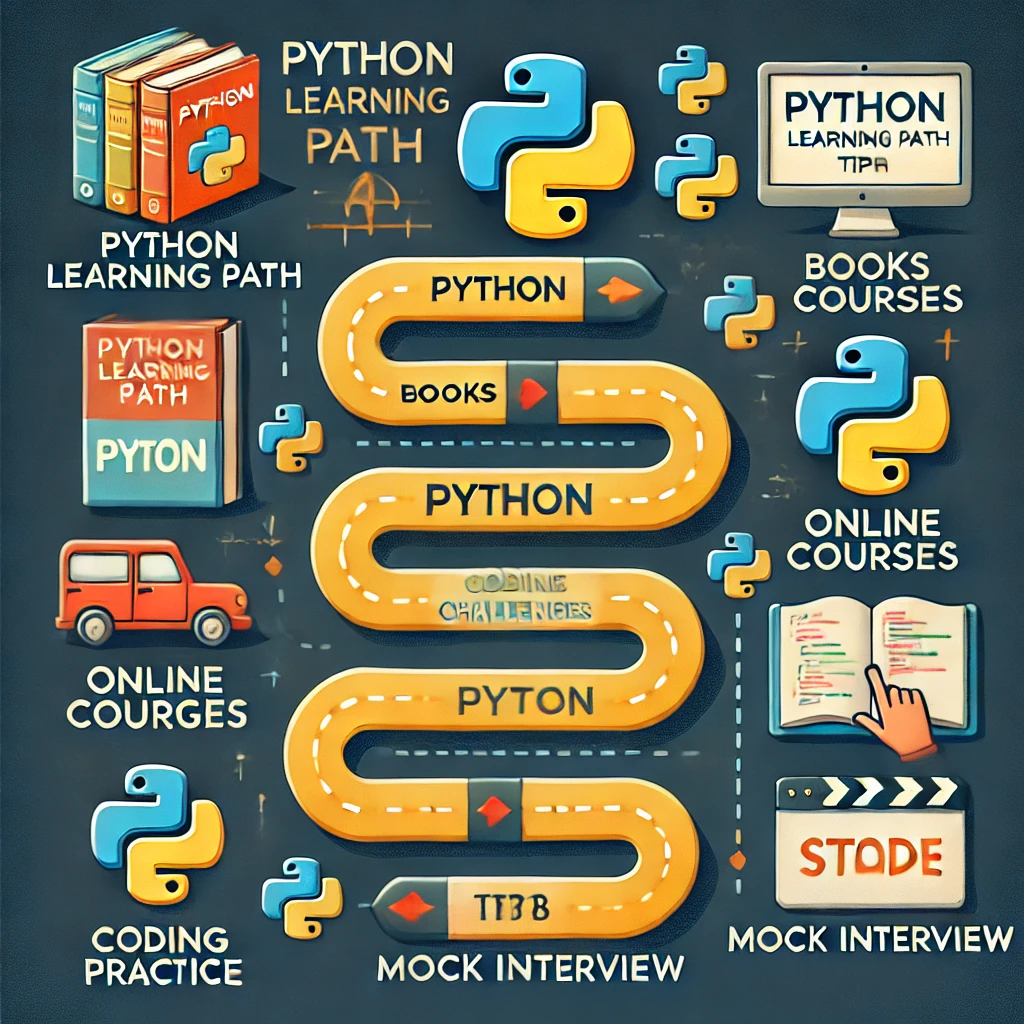
1. Why is Python growing in AI, Blockchain, and Automation? (Backed by Industry Reports)
Python is the #1 language for AI, Blockchain, and Automation due to its simplicity, flexibility, and vast libraries.
Growth Stats (2025 Projections):
- AI & ML: Python dominates 60% of AI projects worldwide. (Source: Gartner)
- Blockchain: Used in Smart Contracts (Hyperledger Fabric, Ethereum). (Source: Deloitte)
- Automation & RPA: Powers 80% of automation scripts in enterprises. (Source: Forrester)
Why Python?
- AI & ML: TensorFlow, PyTorch, Scikit-learn.
- Blockchain: Web3.py, Solidity integration.
- Automation: Selenium, PyAutoGUI, Ansible.
Further Reading: Python in AI & Blockchain
2. What is the future of Python for Web Development?
Python is expected to remain a top choice for web development due to its frameworks and scalability.
Web Development Trends (2025):
- Django & Flask for scalable, secure applications.
- FastAPI gaining traction for high-performance APIs.
- AI-Powered Web Apps using GPT-based chatbots, recommendation engines.
Example (FastAPI for Modern Web Apps):
python
Copy code
fromfastapi
importFastAPI
app = FastAPI()
@app.get("/")
defread_root():
return
{
"message":
"Hello, FastAPI!"}
3. How is Python being used in Cloud Computing?
Python is widely used in AWS, Google Cloud, and Azure for cloud automation, DevOps, and AI-driven cloud applications.
Python in Cloud Computing:
- AWS Lambda, Google Cloud Functions (Serverless Python).
- Infrastructure as Code (IaC) via Terraform & Ansible.
- Cloud AI & ML with TensorFlow on Cloud GPUs.
Example (Python for AWS Automation):
python
Copy code
import boto3
# AWS SDK for Python
s3 = boto3.client(
"s3")
buckets = s3.list_buckets()
([bucket[
"Name"]
forbucket
inbuckets[
"Buckets"]])
Further Reading: Python in Cloud Computing
4. What new features are expected in Python 3.13? (Official Python Roadmap)
Python 3.13 (expected in late 2025) brings performance boosts and new features.
Upcoming Features (Python 3.13 Highlights):
- Better Performance: Faster execution with adaptive interpreter optimizations.
- Pattern Matching Enhancements: Improved
match
case for structured data. - Enhanced Typing Support: Stronger type hints for better debugging.
- Improved Memory Management: Lower memory usage for large applications.
Official Roadmap: Python 3.13 Development
5. Is Python still worth learning in 2025? (Market Demand Insights)
Absolutely! Python remains one of the most in-demand skills globally.
Why Learn Python in 2025?
- Top 3 Programming Languages worldwide (Stack Overflow Developer Survey 2024).
- High-paying jobs: Avg salary $120K+ for Python developers (Glassdoor).
- Multi-Domain Use: AI, Web, Cloud, Blockchain, Automation, IoT.
Example Career Paths with Python:
- AI Engineer → TensorFlow, PyTorch
- Data Scientist → Pandas, NumPy, Scikit-learn
- Cloud Engineer → AWS, GCP, Azure SDKs
Further Reading: Python Job Trends 2025
Conclusion – How to Prepare for a Python Interview?
Cracking a Python interview requires the right strategy, structured learning, and consistent practice. Below are key resources and best practices to help you succeed.
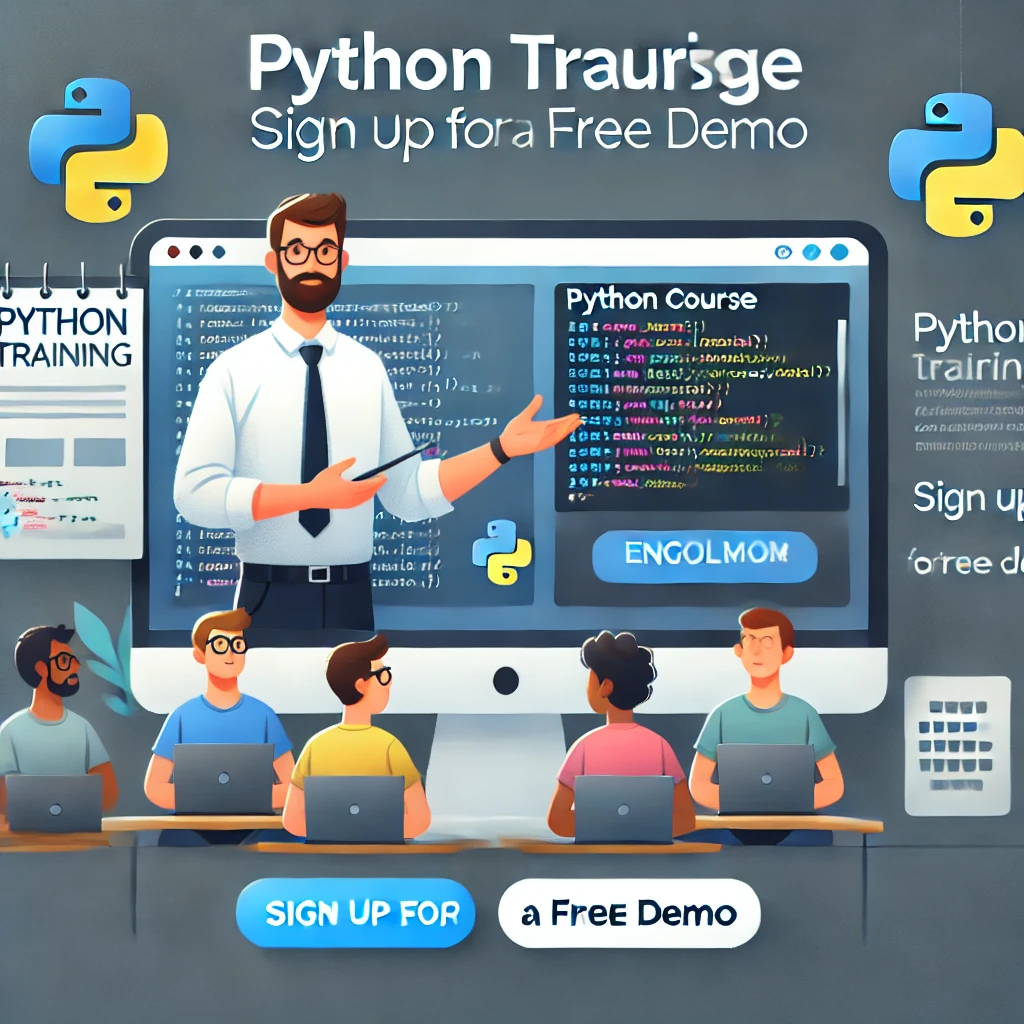
1. Best Python Books, Courses, and Online Resources
Top Books to Master Python:
- Automate the Boring Stuff with Python – Best for beginners.
- Python Crash Course – Hands-on coding exercises.
- Fluent Python – Advanced concepts for experienced developers.
Recommended Python Courses:
- Python Course in Coimbatore – Expert-led training with real-world projects.
- CS50’s Introduction to Python (HarvardX) – Free online course.
- Google’s Python Class – Ideal for beginners.
Further Reading: Python Learning Path
2. How to Practice Python Coding Challenges Effectively?
Follow these steps to improve your coding skills:
- Master Basics: Practice lists, loops, functions, OOP.
- Solve Easy Problems First: Build confidence with simple exercises.
- Focus on Data Structures & Algorithms: Learn sorting, recursion, dynamic programming.
- Write Optimized Code: Use Pythonic solutions with time/space complexity analysis.
- Simulate Real Interviews: Use online coding platforms & mock tests.
Practice Here: Leetcode Python Challenges
3. Top Websites for Python Interview Preparation
📌 Best Platforms to Crack Python Interviews:
- Leetcode – Best for DSA & coding problems.
- GeeksforGeeks – Covers theory + coding exercises.
- HackerRank – Beginner to advanced challenges.
- CodeWars – Python coding exercises with solutions.
4️⃣ Common Mistakes to Avoid in Python Interviews
❌ Using Lists Instead of Generators – Leads to high memory usage.
✅ Use yield
in functions for better performance.
❌ Ignoring Time & Space Complexity – Always optimize your code.
✅ Use built-in functions (sum()
, sorted()
) instead of manual loops.
❌ Not Handling Edge Cases in Coding Problems
✅ Always check for null values, empty lists, negative numbers.
❌ Forgetting to Use Pythonic Syntax
✅ Use list comprehensions, lambda functions, f-strings for clean code.
Further Reading: Python Best Practices